 |
 | NuSOAP and HTTPS | 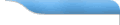 |
Joined: 31 Mar 2008 |
Posts: 4 |
|
|
 |
Posted: Sun Mar 30, 2008 4:00 pm |
|
 |
 |
 |
 |
Hi, I discovered a bug with NuSoap. It works fine with the first HTTPS connection, but after that it keeps using HTTP instead of using HTTPS. Here is my code:
<?php
require_once('lib/nusoap.php');
$proxyhost = isset($_POST['proxyhost']) ? $_POST['proxyhost'] : '';
$proxyport = isset($_POST['proxyport']) ? $_POST['proxyport'] : '';
$proxyusername = isset($_POST['proxyusername']) ? $_POST['proxyusername'] : '';
$proxypassword = isset($_POST['proxypassword']) ? $_POST['proxypassword'] : '';
$client = new nusoap_client('https://myserver/soap/CreateAccountSubscription?wsdl', true, $proxyhost, $proxyport, $proxyusername, $proxypassword);
$err = $client->getError();
if ($err) {
echo '<h2>Constructor error</h2><pre>' . $err . '</pre>';
}
$result = $client->call('CreateAccountSubscription', array(
'password' => 'test',
'username'=>'test',
'accountId'=>'jaimitoc30@hotmail.com',
'description'=>'This is a test',
'maxAccounts'=>"-1",
'msisdn'=>'50764300473',
'product'=>'1',
'provisioningId'=>'1',
'subscriptionType'=>'1'));
if ($client->fault) {
echo '<h2>Fault</h2><pre>'; print_r($result); echo '</pre>';
} else {
$err = $client->getError();
if ($err) {
echo '<h2>Error</h2><pre>' . $err . '</pre>';
} else {
echo '<h2>Result</h2><pre>'; print_r($result); echo '</pre>';
}
}
echo '<h2>Request</h2><pre>' . htmlspecialchars($client->request, ENT_QUOTES) . '</pre>';
echo '<h2>Response</h2><pre>' . htmlspecialchars($client->response, ENT_QUOTES) . '</pre>';
echo '<h2>Debug</h2><pre>' . htmlspecialchars($client->debug_str, ENT_QUOTES) . '</pre>';
?> |
Now, as you can see here is the debug response from the server:
Error
HTTP Error: Couldn't open socket connection to server http://myserver/soap/CreateAccountSubscription, Error (10060): A connection attempt failed because the connected party did not properly respond after a period of time, or established connection failed because connected host has failed to respond.
Request
Response
Debug
2008-03-31 16:54:19.625573 nusoap_client: ctor wsdl=1 timeout=0 response_timeout=30
endpoint=string(72) "https://myserver/soap/CreateAccountSubscription?wsdl"
2008-03-31 16:54:19.838148 nusoap_client: will use lazy evaluation of wsdl from https://myserver/soap/CreateAccountSubscription?wsdl
2008-03-31 16:54:19.868440 nusoap_client: call: operation=CreateAccountSubscription, namespace=http://tempuri_org, soapAction=, rpcParams=, style=rpc, use=encoded, endpointType=wsdl
params=array(9) {
["password"]=>
string(4) "test"
["username"]=>
string(4) "test"
["accountId"]=>
string(22) "jaimitoc30@hotmail.com"
["description"]=>
string(14) "This is a test"
["maxAccounts"]=>
string(2) "-1"
["msisdn"]=>
string(11) "50764300473"
["product"]=>
string(1) "1"
["provisioningId"]=>
string(1) "1"
["subscriptionType"]=>
string(1) "1"
}
headers=bool(false)
2008-03-31 16:54:19.868779 nusoap_client: instantiating wsdl class with doc: https://myserver/soap/CreateAccountSubscription?wsdl
2008-03-31 16:54:19.869091 wsdl: ctor wsdl= timeout=0 response_timeout=30
2008-03-31 16:54:19.869345 wsdl: parse and process WSDL path=
2008-03-31 16:54:19.909125 wsdl: setCredentials username= authtype= certRequest=
array(0) {
}
2008-03-31 16:54:19.909449 wsdl: parse and process WSDL path=https://myserver/soap/CreateAccountSubscription?wsdl
2008-03-31 16:54:19.909693 wsdl: parse WSDL at path=https://myserver/soap/CreateAccountSubscription?wsdl
2008-03-31 16:54:19.927679 wsdl: getting WSDL http(s) URL https://myserver/soap/CreateAccountSubscription?wsdl
2008-03-31 16:54:19.928012 soap_transport_http: ctor url=https://myserver/soap/CreateAccountSubscription?wsdl use_curl= curl_options:
array(0) {
}
...
2008-03-31 16:54:23.216582 soap_transport_http: parsed URL scheme = http
2008-03-31 16:54:23.216822 soap_transport_http: parsed URL host = myserver
2008-03-31 16:54:23.217056 soap_transport_http: parsed URL path = /soap/CreateAccountSubscription
2008-03-31 16:54:23.217304 soap_transport_http: set header Host: myserver
2008-03-31 16:54:23.217569 soap_transport_http: set header User-Agent: NuSOAP/0.7.3 (1.114)
2008-03-31 16:54:23.217825 soap_transport_http: set header Content-Type: text/xml; charset=ISO-8859-1
2008-03-31 16:54:23.218069 soap_transport_http: set header SOAPAction: ""
2008-03-31 16:54:23.218551 soap_transport_http: entered send() with data of length: 411
2008-03-31 16:54:23.218797 soap_transport_http: connect connection_timeout 0, response_timeout 30, scheme http, host myserver, port 80
2008-03-31 16:54:23.219081 soap_transport_http: calling fsockopen with host myserver connection_timeout 0
2008-03-31 16:55:23.286861 soap_transport_http: Couldn't open socket connection to server http://myserver/soap/CreateAccountSubscription, Error (10060): A connection attempt failed because the connected party did not properly respond after a period of time, or established connection failed because connected host has failed to respond.
2008-03-31 16:55:23.287213 nusoap_client: Error: HTTP Error: Couldn't open socket connection to server http://myserver/soap/CreateAccountSubscription, Error (10060): A connection attempt failed because the connected party did not properly respond after a period of time, or established connection failed because connected host has failed to respond.
As you can see at the beginning of the Debug it connects fine and works fine, however the next time it starts using HTTP instead of HTTPS. Is this a bug, or am I doing something wrong? I think it is a bug since it should always use HTTPS if I specified it.
Any help would be appreciated.
Thank you.
|
|
 |
 | | 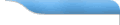 |
 | i understand | 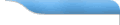 |
 |
 | | 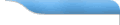 |
 |
 | | 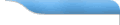 |
 |
 | | 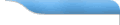 |
 |
 | | 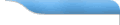 |
Joined: 31 Mar 2008 |
Posts: 4 |
|
|
 |
Posted: Tue Apr 08, 2008 4:46 pm |
|
 |
 |
 |
 |
dmitri wrote: | Would you please provide a _short_ snippet (3-4 lines would be fairly enough!) from your WSDL where the link is defined? |
Well, I can, however let me explain you, the port 80 in the server is closed, meaning I need to use strictly 443 https for al transactions. It does the first transportation fine, however, the next one it changes back to http 80 instead of continue using 443. Here is the WSDL
- <wsdl:service name="CreateAccountSubscriptionServiceIntf">
- <wsdl:port name="CreateAccountSubscriptionServiceIntfHttpPort" binding="tns:CreateAccountSubscriptionServiceIntfHttpBinding">
<wsdlsoap:address location="http://myserver/soap/CreateAccountSubscription" />
Also, I made a little client with java and it worked fine. If you like I can provide both my Java code and my PHP code.
regards and thanks for your time.
|
|
 |
 | | 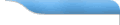 |
You cannot post new topics in this forum You cannot reply to topics in this forum You cannot edit your posts in this forum You cannot delete your posts in this forum You cannot vote in polls in this forum
|
All times are GMT - 5 Hours
Page 1 of 1
|
|
|
|  |