 |
 | Switching from asp to php (Ajax Calls) | 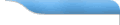 |
Joined: 03 Jan 2008 |
Posts: 15 |
|
|
 |
Posted: Wed Jan 09, 2008 6:34 pm |
|
 |
 |
 |
 |
I'm having a problem. I've got a program in ASP (that works!). I'm moving it to PHP. Basically, I'm using Ajax to go back and forth to the server. I send the request with XML (in the post request) and the PHP code on the server breaks down the XML request, executes it, and returns some XML to display.
So, when I call the server, I never get a return! It's the strangest thing. If I change the script to just output static XML, it comes back just fine, but parsing through the XML seems to stop it from returning. I've tried changing the method of parsing through the XML (I've tried Expat, SimpleXML, DOMDocument...) but it doesn't seem to make a difference! Then, when I change the page, I finally get the callback! (I put an alert in the callback and displayed the status... I get the first callback (status=1), then nothing until I change pages, then I get the rest (2, 3, 4) all in rapid order).
So I used the debugger and I confirmed that the code is being executed on the server, but I still don't get the callback until I change the page.
Am I missing something? Any help is appreciated!!! Thanks.
|
|
 |
 | | 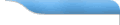 |
 |
 | | 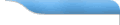 |
Joined: 03 Jan 2008 |
Posts: 15 |
|
|
 |
Posted: Thu Jan 10, 2008 1:37 pm |
|
 |
 |
 |
 |
gilzow wrote: | any chance you can post the code so we can take a look at it? |
As noted above, it doesn't seem to matter HOW the XML is parsed... so right now, I'm using this code to parse it (it parses the XML into an array structure):
http://myserver/software/phpxml
My code is here (The xmlParser.php is the above referenced code):
<? require "../include/common.php"; ?>
<? require "../include/xmlParser.php"; ?>
<?
if (!loggedIn()) {
// just send a 404 if they're not logged in.
header("HTTP/1.0 404 Not Found");
}
else {
header("Content-Type: application/xml; charset=UTF-8");
// $xmlDoc = new SimpleXMLElement(getRequestBody());
//$xmlDoc = new DOMDocument();
$req = getRequestBody();
$xmlDoc = XML_unserialize($req);
//print_r($xmlDoc);
if (empty($xmlDoc['Configuration'])) {
header("HTTP/1.0 500 Error on Server");
}
else {
$entries = $xmlDoc['Configuration'];
echo "<Configuration>" . CRLF;
foreach ($entries as $entry) {
$eName = $entry["Name"];
$eValue = $entry["Value"];
setValue($eName, $eValue);
echo " <ENTRY>" . CRLF;
echo " <Name>" . $eName . "</Name>" . CRLF;
echo " <Value>" . $eValue . "</Value>" . CRLF;
echo " </ENTRY>" . CRLF;
}
echo "</Configuration>" . CRLF;
}
}
?>
|
Now, as noted before, if I just statically output some XML, it seems to work just fine.
|
|
 |
 | | 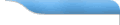 |
Joined: 10 Feb 2004 |
Posts: 93 |
|
|
 |
Posted: Thu Jan 10, 2008 2:55 pm |
|
 |
 |
 |
 |
If you go to the script directly, do you see the XML?
You might also try adding header ("content-type: text/xml"); |
before you begin echo'ing the XML. I usually go with JSON instead of XML, but I've had a couple of situations where things didnt work properly until I added an appropriate header.
|
|
Joined: 03 Jan 2008 |
Posts: 15 |
|
|
 |
Posted: Thu Jan 10, 2008 3:07 pm |
|
 |
 |
 |
 |
gilzow wrote: | If you go to the script directly, do you see the XML?
You might also try adding header ("content-type: text/xml"); |
before you begin echo'ing the XML. I usually go with JSON instead of XML, but I've had a couple of situations where things didnt work properly until I added an appropriate header. |
I can echo the XML just fine. The problem is that when I parse the incoming XML, it never seems to return.
I'd be willing to try JSON... Can you point me to a good PHP implementation of this?
|
|
 |
 | | 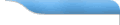 |
Joined: 10 Feb 2004 |
Posts: 93 |
|
|
 |
Posted: Thu Jan 10, 2008 3:39 pm |
|
 |
 |
 |
 |
JudoDan wrote: | gilzow wrote: | If you go to the script directly, do you see the XML?
You might also try adding header ("content-type: text/xml"); |
before you begin echo'ing the XML. I usually go with JSON instead of XML, but I've had a couple of situations where things didnt work properly until I added an appropriate header. |
I can echo the XML just fine. The problem is that when I parse the incoming XML, it never seems to return. |
I think I'm a bit confused here... because you are sending XML from the client, parsing it on the server side, then formulating new XML on the server-side, sending that XML back to the client, and then finally parsing it on the client-side, I'm unsure as to which side (client or server) is failing to parse. If you can echo the parsed XML just fine, then I will assume that the javascript is not aware that what it has received is truly XML. The header should help that.
If I have misunderstood you, and the php (server-side) is failing to parse through the XML it has received, then it might be with how you are testing your $xmlDoc['Configuration']. Instead of if (empty($xmlDoc['Configuration'])) {
header("HTTP/1.0 500 Error on Server");
} |
try doing if(is_array($xmlDoc['Configuration']) && count($xmlDoc['Configuration']) != 0){
//iterate through your array
} else {
header("HTTP/1.0 500 Error on Server");
} |
As it is possible your $xmlDoc['Configuration'] isnt empty, but also isnt an array.
JudoDan wrote: | I'd be willing to try JSON... Can you point me to a good PHP implementation of this? |
http://pear.php.net/pepr/pepr-proposal-show.php?id=198 is the one I've been using and have been quite pleased.
|
|
 |
 | | 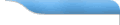 |
Joined: 03 Jan 2008 |
Posts: 15 |
|
|
 |
Posted: Thu Jan 10, 2008 4:35 pm |
|
 |
 |
 |
 |
gilzow wrote: | JudoDan wrote: | gilzow wrote: | If you go to the script directly, do you see the XML?
You might also try adding header ("content-type: text/xml"); |
before you begin echo'ing the XML. I usually go with JSON instead of XML, but I've had a couple of situations where things didnt work properly until I added an appropriate header. |
I can echo the XML just fine. The problem is that when I parse the incoming XML, it never seems to return. |
I think I'm a bit confused here... because you are sending XML from the client, parsing it on the server side, then formulating new XML on the server-side, sending that XML back to the client, and then finally parsing it on the client-side, I'm unsure as to which side (client or server) is failing to parse. If you can echo the parsed XML just fine, then I will assume that the javascript is not aware that what it has received is truly XML. The header should help that.
If I have misunderstood you, and the php (server-side) is failing to parse through the XML it has received, then it might be with how you are testing your $xmlDoc['Configuration']. Instead of if (empty($xmlDoc['Configuration'])) {
header("HTTP/1.0 500 Error on Server");
} |
try doing if(is_array($xmlDoc['Configuration']) && count($xmlDoc['Configuration']) != 0){
//iterate through your array
} else {
header("HTTP/1.0 500 Error on Server");
} |
As it is possible your $xmlDoc['Configuration'] isnt empty, but also isnt an array.
|
I think I'm not explaining this properly. I'm sending XML from the client to the server. The server parses it (This is just the first piece of a complicated system...) and returns XML. Currently, I have it working perfectly (for over a year) in ASP. The javascript hasn't changed. The problem is, I make the call to the server and it doesn't return to the client until I change pages (strange, doesn't matter how long I wait). When I debug it, I can confirm that the code is executing properly, but when it exits, it never returns to the client. If I comment out the code that parses the XML and just return some static XML, it works perfectly. I've tried THREE different ways of parsing the XML (and confirmed it worked properly using the debugger) and it never returns. This is the most bizarre thing I've seen.
On the JS side, I put alerts in the callback and confirmed it wasn't getting back to the client.
Thanks, will check it out. I am also passing HTML code (It's a CMS) back and forth... Is that a problem with JSON? (Never used it...)
|
|
 |
 | | 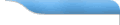 |
 |
 | | 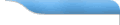 |
 |
 | | 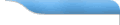 |
Joined: 03 Jan 2008 |
Posts: 15 |
|
|
 |
Posted: Thu Jan 10, 2008 6:12 pm |
|
 |
 |
 |
 |
gilzow wrote: | JudoDan wrote: | As noted... I have stepped through this code with the debugger and it is working as expected. |
if you can look at the output directly and it appears correct, then, without seeing it actually in use, I'm as baffled as you. I've done tons with php+ajax and have never run into what you are describing. sorry i wasnt able to help you more. |
Yes, it's F'ing baffling!!!!
But I might switch to sending data to the server using JSON to see if it changes anything...
|
|
You cannot post new topics in this forum You cannot reply to topics in this forum You cannot edit your posts in this forum You cannot delete your posts in this forum You cannot vote in polls in this forum
|
All times are GMT - 5 Hours
Page 1 of 2
|
|
|
|  |